728x90
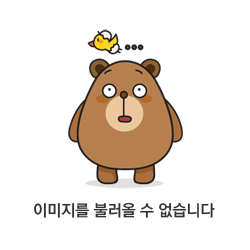
이번 포스팅에서 다뤄볼 Vue.js 어디서든 Store 호출하기입니다.
store.js
import { createStore } from "vuex"
const store = createStore({
state(){
return{
data : 'Vuex'
}
},
mutations : {
setDataFunction(context, text){
context.data = text
}
},
actions : {
setTimeOutFunction(context, text){
setTimeout( text => {
this.commit('setDataFunction', text)
}, 3000)
}
},
export default store
Actions 함수에서 Mutations 함수를 호출할때 사용하는 구문입니다.
this.commit('Mutations메소드명', 매개변수)
this.commit('Mutations메소드명', {매개변수1, 매개변수2})
this.commit() 구문을 이용하여 호출 할 수 있습니다.
Mutations 함수에서 Actions 함수를 호출할 때 사용하는 구문입니다.
this.dispatch('Actions메소드명', 매개변수)
this.dispatch('Actions메소드명', {매개변수1, 매개변수2})
위의 예제처럼 사용할 수 있습니다.
Store 내부적인 호출은 단순 this.commit(), this.dispatch()를 사용하지만
하지만 일반 컴포넌트에서 호출하려면 미리 선언을 해야 하는 제약조건이 있습니다.
제약조건으로는... mapMutations와... mapActions에 선언을 하는 것입니다.
<template>
<button @click="setDataFunction('여기는 Mutations함수')">버튼1</button>
<button @click="setTimeOutFunction('여기는 Actions함수')">버튼2</button>
<inpout type="text" size="30" v-model="data"></input>
</template>
<script>
import {mapMutations, mapActions, ...mapState} from 'vuex'
export default {
name: 'App',
// this.$store.state.data로 값을 조회할 수 있지만 코드 간결화를 위해 ...mapState에 축약어를 선언하였습니다.
computed : {
...mapState({
data : state => state.data,
})
},
methods : {
...mapMutations({
// 단일 store의 경우 namespaced를 설정하지않기 때문에 메소드명만 호출하시면 됩니다.
setDataFunction : 'setDataFunction',
// 만약 모듈화된 store의 경우에는 namespaced : true 값을 주기 때문에 메소드명 앞에 해당 store명을 기재합니다.
// setDataFunction : 'storeName/setDataFunction'
}),
...mapActions({
setTimeOutFunction : 'setTimeOutFunction',
// setTimeOutFunction : 'storeName/setTimeOutFunction'
}),
},
}
</script>
<style scoped>
...
</style>
위의 코드와 같이 main.js에서 선언된 store.js 내부의 동기 함수와 비동기 함수를 호출하는 방법에 대해 알 수 있습니다.
이번에는 Router에서도 store를 사용해보겠습니다.
(Router란?)
// Router 포스팅 주소
(Axios란??)
// Axios 포스팅 주소
import { createRouter } from "vue-router"
import store from './store.js'
import axios from 'axios'
// 간단한 token 인증방식의 router입니다.
const roleCheck = (repData) => {
axios({
url: repData.url,
method: repData.method,
data: repData.data
}).then(res => {
if(res.data === 'ok') {
// store.commit()을 이용해 Mutattions 메소드를 호출합니다
store.commit("setDataFunction", '로그인 완료된 사용자입니다.')
// store.dispatch() 메소드를 이용하여 비동기 store 함수를 호출할 수 있지만
// 비동기 함수 내부의 비동기 함수 호출은 선호하지 않는 방식이여서 넘어가겠습니다!.
} else {
repData.next(repData.falsePath)
}
}).catch(() => {
repData.next(repData.falsePath)
})
}
const routes = [
{
path : '/',
component : Home,
},
{
path : '/login',
component : Login,
},
{
path : '/main',
component : Main,
beforeEnter: (to, from, next) => {
const repData = {
url : '/token/check',
method : 'post',
falsePath: '/',
next: next,
data: {
token : sessionStorage.getItem("token")
}
}
roleCheck(repData)
}
},
]
const router = createRouter({
routes
})
export default router;
위의 예제처럼 간단한 router 로그인 도중에 store의 메서드에 접근할 수 있습니다.
다음 포스팅에서는 Axios에 대해 알아보겠습니다.
728x90
'Frontend > Vue.Js' 카테고리의 다른 글
[Vue] Vuex 란? ( npm, 기본 구문 ) (0) | 2022.01.08 |
---|---|
[Vue] 다양한 디렉티브(v-땡땡)을 사용하자! (0) | 2022.01.07 |
[Vue] Vue.js란? view? vue? (0) | 2022.01.07 |